Building a Valentine's Day Page Creator with Next.js
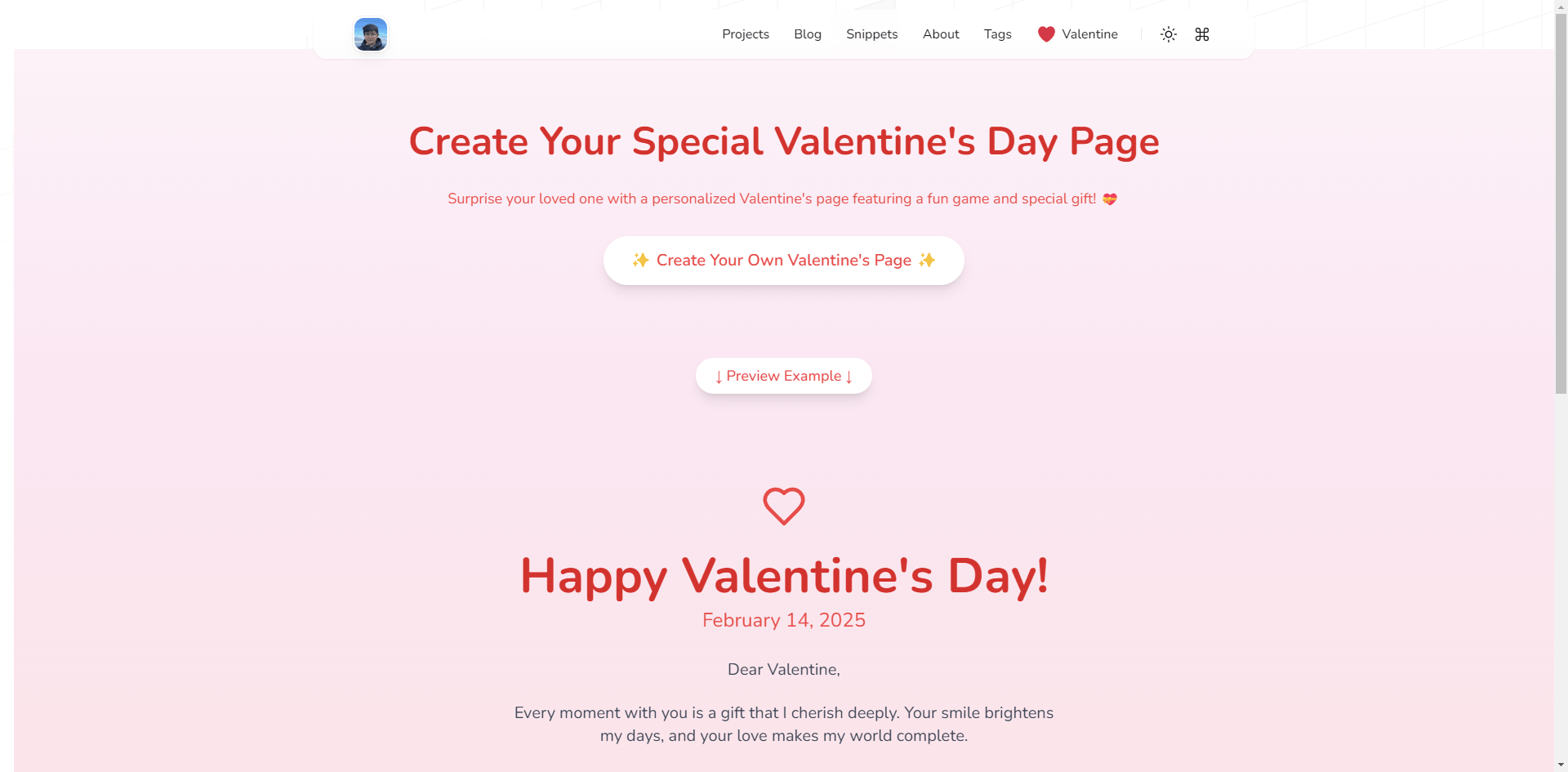
- Published on
- /4 mins read/---
Introduction
Recently, I implemented a Valentine's Day page creator feature that allows users to create personalized Valentine's pages with customizable games and messages. In this post, I'll share the technical details of how this system was built, including the database design, API implementation, and frontend architecture.
System Architecture
The system consists of three main components:
- Page Creator Interface: A form-based UI for customizing Valentine's pages
- API Layer: Handles page creation and retrieval
- Database Layer: Stores page configurations and settings
Database Schema
The valentine configuration is stored in a MongoDB collection with the following structure:
interface ValentineConfig {
recipient: string
sender: string
message: string
gameSettings: {
duration: number // Game duration in seconds
targetScore: number // Required score to win
customEmojis?: {
// Optional custom emojis
player?: string
target?: string
}
}
language: 'en' | 'zh' // Support for multiple languages
createdAt: Date
}
API Implementation
The API routes handle page creation and retrieval:
// POST /api/valentine
export async function POST(req: Request) {
try {
const body = await req.json()
const config = await validateConfig(body)
const record = await db.valentine.create({
config,
createdAt: new Date(),
})
return Response.json({ id: record.id })
} catch (error) {
return Response.json({ error: 'Failed to create page' }, { status: 500 })
}
}
// GET /api/valentine?id={id}
export async function GET(req: Request) {
const id = new URL(req.url).searchParams.get('id')
if (!id) {
return Response.json({ error: 'Missing id' }, { status: 400 })
}
const record = await getValentineConfig(id)
if (!record) {
return Response.json({ error: 'Not found' }, { status: 404 })
}
return Response.json(record)
}
Page Creation Flow
The page creation process follows these steps:
User fills out the creation form with:
- Recipient name
- Personal message
- Game settings
- Language preference
Form data is validated and sent to the API
API creates a new record and returns a unique ID
User gets a shareable link to their Valentine's page
export default function CreatePage() {
const [config, setConfig] = useState<ValentineConfig>({
recipient: '',
sender: '',
message: '',
gameSettings: {
duration: 20,
targetScore: 10,
},
language: 'en',
})
const handleSubmit = async (e: React.FormEvent) => {
e.preventDefault()
const response = await fetch('/api/valentine', {
method: 'POST',
body: JSON.stringify(config),
})
const { id } = await response.json()
// Redirect to the created page
router.push(`/valentine/${id}`)
}
return <form onSubmit={handleSubmit}>{/* Form fields */}</form>
}
Dynamic Page Generation
Each Valentine's page is dynamically generated based on the stored configuration:
export default async function Page({ params }: { params: { id: string } }) {
const { id } = params
const record = await getValentineConfig(id)
if (!record) {
return notFound()
}
return <ValentinePageContent config={record.config} />
}
Performance Considerations
Several optimizations were implemented:
- Database Indexing: Created indexes on frequently queried fields
- Caching: Implemented caching for page configurations
- Validation: Added robust input validation to prevent invalid data
- Error Handling: Comprehensive error handling throughout the system
Future Improvements
Potential enhancements for the future:
- Analytics: Track page views and interactions
- More Customization: Additional game types and themes
- Social Sharing: Better social media integration
- Preview Mode: Allow users to preview before sharing
Conclusion
Building this Valentine's Day page creator was an interesting challenge that combined several aspects of modern web development. The system provides a fun and interactive way for users to create personalized Valentine's messages while maintaining good performance and reliability.
The combination of Next.js, TypeScript, and MongoDB provided a solid foundation for building this feature, while careful attention to user experience and performance ensured a smooth creation process.